All Types GNIIT/NIIT Solution (Mr Jerry) Follow me on G+ and Twitter for more updates Twitter @mrjerry0001 #Write for us and get paid contact us On hike App:- @mrjerry4968
Pages
- Home
- HTML LAB@HOME
- DSA LAB@HOME
- WCD LAB@HOME
- C# LAB@HOME
- RDBMS LAB@HOME
- IADRT Lab@home
- EBDD LAB@HOME
- ACPC Lab@home
- LBEPS Lab@home
- ICTWC LAB@HOME
- SQL LAB@HOME
- WINDOWS APP LAB@HOME
- JAVA LAB@HOME
- NETWORK ESSENTIALS + LAB@HOME
- ASP.NET LAB@HOME
- LBEPS CYCLE TEST
- ASP.NET CYCLE TEST
- JAVA CYCLE TEST
- RDBMS CYCLE TEST
- DSA CYCLE TEST
- NIIT-HTML CYCLE TEST
- NIIT ASSIGNMENTS
- NIIT PROJECTS
- CORE JAVA NOTES
- NIIT BOOKS
- NIIT MT SOLVED
Sunday 14 May 2017
Java Applet ~ mkniit
Java Legacy Classes ~ mkniit
Legacy Classes
Early version of java did not include the Collection framework. It only defined several classes and interface that provide method for storing objects. When Collection framework were added in J2SE 1.2, the original classes were reengineered to support the collection interface. These classes are also known as Legacy classes. All legacy claases and interface were redesign by JDK 5 to support Generics.
The following are the legacy classes defined by java.util package
- Dictionary
- HashTable
- Properties
- Stack
- Vector
There is only one legacy interface called Enumeration
NOTE: All the legacy classes are syncronized
Java Comparator Interface ~ mkniit
In Java, Comparator interface is used to order the object in your own way. It gives you ability to decide how element are stored within sorted collection and map.
Comparator Interface defines
compare()
method. This method compare two object and return 0 if two object are equal. It returns a positive value if object1 is greater than object2. Otherwise a negative value is return. The method can throw a ClassCastException if the type of object are not compatible for comparison.Example
Student class
class Student int roll; String name; Student(int r,String n) { roll = r; name = n; } public String toString() { return roll+" "+name; }
Java Map Interface ~ mkniit
A Map stores data in key and value association. Both key and values are objects. The key must be unique but the values can be duplicate. Although Maps are a part of Collection Framework, they can not actually be called as collections because of some properties that they posses. However we can obtain a collection-view of maps.
Interface | Description |
---|---|
Map | Maps unique key to value. |
Map.Entry | Describe an element in key and value pair in a map. This is an inner class of map. |
NavigableMap | Extends SortedMap to handle the retrienal of entries based on closest match searches |
SortedMap | Extends Map so that key are maintained in an ascending order. |
Java Iterator and ListIterator ~ mkniit
Accessing a Collection
To access, modify or remove any element from any collection we need to first find the element, for which we have to cycle throught the elements of the collection. There are three possible ways to cycle through the elements of any collection.
- Using Iterator interface
- Using ListIterator interface
- Using for-each loop
Accessing elements using Iterator
Iterator Interface is used to traverse a list in forward direction, enabling you to remove or modify the elements of the collection. Each collection classes provide iterator() method to return an iterator.
Java Collection Classes ~ mkniit
The Collection classes
Java provides a set of Collection classes that implements Collection interface. Some of these classes provide full implementations that can be used as it is and other abstract classes provides skeletal implementations that can be used as starting points for creating concrete collections.
ArrayList class
- ArrayList class extends AbstractList class and implements the List interface.
- ArrayList supports dynamic array that can grow as needed. ArrayList has three constructors.
ArrayList() ArrayList( Collection C ) ArrayList( int capacity )
- ArrayLists are created with an initial size, when this size is exceeded, it gets enlarged automatically.
- It can contain Duplicate elements and maintains the insertion order.
Java Collection Interfaces ~ mkniit
Interfaces of Collection Framework
The collection framework has a lot of Interfaces, setting the fundamental nature of various collection classes. Lets study the most important Interfaces in the collection framework.
The Collection Interface
- It is at the top of collection heirarchy and must be implemented by any class that defines a collection. Its general declaration is,
interface Collection < E >
- Following are some of the commonly used methods in this interface.
Methods Description add( E obj ) Used to add objects to a collection. Doesn't add duplicate elements to the collection. addAll( Collection C ) Add all elements of collection C to the invoking collection remove( Object obj ) To remove an object from collection removeAll( Collection C ) Removes all element of collection C from the invoking collection contains( Object obj ) To determine whether an object is present in collection or not isEmpty() Returns true if collection is empty, else returns false size() returns number of elements present in collection
Java Introduction to Collection ~ mkniit
Collection Framework
Collection framework was not part of original Java release. Collections was added to J2SE 1.2. Prior to Java 2, Java provided adhoc classes such as Dictionary, Vector, Stack and Properties to store and manipulate groups of objects. Collection framework provides many important classes and interfaces to collect and organize group of alike objects.
Important Interfaces of Collection API
Interface | Description |
---|---|
Collection | Enables you to work with groups of object; it is at the top of collection hierarchy |
Deque | Extends queue to handle double ended queue. |
List | Extends collection to handle sequences list of object. |
Queue | Extends collection to handle special kind of list in which element are removed only from the head. |
Set | Extends collection to handle sets, which must contain unique element. |
SortedSet | Extends sets to handle sorted set. |
Java Generics ~ mkniit
A class or interface that operates on parameterized type is called Generic. Generics was first introduced in Java5. Now it is one of the most profound feature of java programming language. It provides facility to write algorithm independent of any specific type of data. Generics also provide type safety.
Using Generics, it becomes possible to create a single class or method that automatically works with all types of data(Integer, String, Float etc). It expanded the ability to reuse code safely and easily.
Example of Generic class
class Gen <T> { T ob; //an object of type T is declared Gen(T o) //constructor { ob = o; } public T getOb() { return ob; } } class Test { public static void main (String[] args) { Gen < Integer> iob = new Gen(100); //instance of Integer type Gen Class. int x = iob.getOb(); System.out.print in(x); Gen < String> sob = new Gen ("Hello"); //instance of String type Gen Class. String str = sob.getOb(); } }
Java Java Networking ~ mkniit
Networking in Java
Java is a premier language for network programming. java.net package encapsulate large number of classes and interface that provides an easy-to use means to access network resources. Here are some important classes and interfaces of java.net package.
Some Important Classes
CLASSES | |
---|---|
CacheRequest | CookieHandler |
CookieManager | Datagrampacket |
Inet Address | ServerSocket |
Socket | DatagramSocket |
Proxy | URL |
URLConnection |
Java Serialization ~ mkniit
Serialization and Deserialization in Java
Serialization is a process of converting an object into a sequence of bytes which can be persisted to a disk or database or can be sent through streams. The reverse process of creating object from sequence of bytes is called deserialization.
A class must implement Serializable interface present in java.io package in order to serialize its object successfully. Serializable is a marker interface that adds serializable behaviour to the class implementing it.
Java provides Serializable API encapsulated under java.io package for serializing and deserializing objects which include,
- java.io.serializable
- java.io.Externalizable
- ObjectInputStream
- and ObjectOutputStream etc.
Java I/O Stream ~ mkniit
IO Stream
Java performs I/O through Streams. A Stream is linked to a physical layer by java I/O system to make input and output operation in java. In general, a stream means continuous flow of data. Streams are clean way to deal with input/output without having every part of your code understand the physical.
Java encapsulates Stream under java.io package. Java defines two types of streams. They are,
- Byte Stream : It provides a convenient means for handling input and output of byte.
- Character Stream : It provides a convenient means for handling input and output of characters. Character stream uses Unicode and therefore can be internationalized.
Java Autoboxing and Unboxing ~ mkniit
type wrapper
Java uses primitive types such as int, double or float to hold the basic data types for the sake of performance. Despite the performance benefits offered by the primitive types, there are situation when you will need an object representation. For example, many data structures in Java operate on objects, so you cannot use primitive types with those data structures. To handle these situations Java provides type Wrappers which provide classes that encapsulate a primitive type within an object.
- Character : It encapsulates primitive type char within object.
Character (char ch)
- Boolean : It encapsulates primitive type boolean within object.
Java Enumerations ~ mkniit
Enumerations was added to Java language in JDK5. Enumeration means a list of named constant. In Java, enumeration defines a class type. An Enumeration can have constructors, methods and instance variables. It is created using enum keyword. Each enumeration constant is public, static and final by default. Even though enumeration defines a class type and have constructors, you do not instantiate an enum using new. Enumeration variables are used and declared in much a same way as you do a primitive variable.
How to Define and Use an Enumeration
- An enumeration can be defined simply by creating a list of enum variable. Let us take an example for list of Subject variable, with different subjects in the list.
enum Subject //Enumeration defined { Java, Cpp, C, Dbms }
- Identifiers Java, Cpp, C and Dbms are called enumeration constants. These are public, static final by default.
Java Interthread Communication ~ mkniit
Java provide benefit of avoiding thread pooling using interthread communication. The wait(), notify(), notifyAll() of Object class. These method are implemented as final in Object. All three method can be called only from within a synchronized context.
- wait() tells calling thread to give up monitor and go to sleep until some other thread enters the same monitor and call notify.
- notify() wakes up a thread that called wait() on same object.
- notifyAll() wakes up all the thread that called wait() on same object.
java Synchronization ~ mkniit
At times when more than one thread try to access a shared resource, we need to ensure that resource will be used by only one thread at a time. The process by which this is achieved is called synchronization. The synchronization keyword in java creates a block of code referred to as critical section.
Every Java object with a critical section of code gets a lock associated with the object. To enter critical section a thread need to obtain the corresponding object's lock.
General Syntax :
synchronized (object) { //statement to be synchronized }
Java Joining a thread ~ mkniit
Joining threads
Sometimes one thread needs to know when another thread is ending. In java, isAlive() and join() are two different methods to check whether a thread has finished its execution.
The isAlive() method returns true if the thread upon which it is called is still running otherwise it returns false.
final boolean isAlive()
But, join() method is used more commonly than isAlive(). This method waits until the thread on which it is called terminates.
final void join() throws InterruptedException
Using join() method, we tell our thread to wait until the specified thread completes its execution. There are overloaded versions of join() method, which allows us to specify time for which you want to wait for the specified thread to terminate.
Java Creating a thread ~ mkniit
Java defines two ways by which a thread can be created.
- By implementing the Runnable interface.
- By extending the Thread class.
Implementing the Runnable Interface
The easiest way to create a thread is to create a class that implements the runnable interface. After implementing runnable interface , the class needs to implement the run() method, which is of form,
public void run()
- run() method introduces a concurrent thread into your program. This thread will end when run() returns.
- You must specify the code for your thread inside run() method.
- run() method can call other methods, can use other classes and declare variables just like any other normal method.
Java Thread class ~ mkniit
Thread class is the main class on which Java's Multithreading system is based. Thread class, along with its companion interface Runnable will be used to create and run threads for utilizing Multithreading feature of Java.
Constructors of Thread class
- Thread ( )
- Thread ( String str )
- Thread ( Runnable r )
- Thread ( Runnable r, String str)
You can create new thread, either by extending Thread class or by implementing Runnable interface. Thread class also defines many methods for managing threads. Some of them are,
Java Multithreading ~ mkniit
Introduction to Multithreading
A program can be divided into a number of small processes. Each small process can be addressed as a single thread (a lightweight process). Multithreaded programs contain two or more threads that can run concurrently. This means that a single program can perform two or more tasks simultaneously. For example, one thread is writing content on a file at the same time another thread is performing spelling check.
In Java, the word thread means two different things.
- An instance of Thread class.
- or, A thread of execution.
An instance of Thread class is just an object, like any other object in java. But a thread of execution means an individual "lightweight" process that has its own call stack. In java each thread has its own call stack.
Java Chained Exceptions ~ mkniit
Chained Exception was added to Java in JDK 1.4. This feature allow you to relate one exception with another exception, i.e one exception describes cause of another exception. For example, consider a situation in which a method throws an ArithmeticException because of an attempt to divide by zero but the actual cause of exception was an I/O error which caused the divisor to be zero. The method will throw only ArithmeticException to the caller. So the caller would not come to know about the actual cause of exception. Chained Exception is used in such type of situations.
Two new constructors and two methods were added to Throwable class to support chained exception.
- Throwable( Throwable cause )
- Throwable( String str, Throwable cause )
In the first form, the paramter cause specifies the actual cause of exception. In the second form, it allows us to add an exception description in string form with the actual cause of exception.
getCause() and initCause() are the two methods added to Throwable class.
- getCause() method returns the actual cause associated with current exception.
- initCause() set an underlying cause(exception) with invoking exception.
Java Method Overriding with Exception Handling ~ mkniit
There are few things to remember when overriding a method with exception handling. If super class method does not declare any exception, then sub class overriden method cannot declare checked exception but it can declare unchecked exceptions.
Example of Subclass overriden Method declaring Checked Exception
import java.io.*;
class Super
{
void show() { System.out.println("parent class"); }
}
public class Sub extends Super
{
void show() throws IOException //Compile time error
{ System.out.println("parent class"); }
public static void main( String[] args )
{
Super s=new Sub();
s.show();
}
}
Java User made Exception Subclass ~ mkniit
User defined Exception subclass
You can also create your own exception sub class simply by extending java Exception class. You can define a constructor for your Exception sub class (not compulsory) and you can override the toString() function to display your customized message on catch.
class MyException extends Exception { private int ex; MyException(int a) { ex=a; } public String toString() { return "MyException[" + ex +"] is less than zero"; } }
Java throw, throws and finally ~ mkniit
throw keyword is used to throw an exception explicitly. Only object of Throwable class or its sub classes can be thrown. Program execution stops on encountering throw statement, and the closest catch statement is checked for matching type of exception.
Syntax :
throw ThrowableInstance
Creating Instance of Throwable class
There are two possible ways to get an instance of class Throwable,
- Using a parameter in catch block.
- Creating instance with new operator.
new NullPointerException("test");
This constructs an instance of NullPointerException with name test.
Example demonstrating throw Keyword
class Test { static void avg() { try { throw new ArithmeticException("demo"); } catch(ArithmeticException e) { System.out.println("Exception caught"); } } public static void main(String args[]) { avg(); } }
In the above example the avg() method throw an instance of ArithmeticException, which is successfully handled using the catch statement.
throws Keyword
Any method capable of causing exceptions must list all the exceptions possible during its execution, so that anyone calling that method gets a prior knowledge about which exceptions to handle. A method can do so by using the throws keyword.
Syntax :
type method_name(parameter_list) throws exception_list { //definition of method }
NOTE : It is necessary for all exceptions, except the exceptions of type Error and RuntimeException, or any of their subclass.
Example demonstrating throws Keyword
class Test { static void check() throws ArithmeticException { System.out.println("Inside check function"); throw new ArithmeticException("demo"); } public static void main(String args[]) { try { check(); } catch(ArithmeticException e) { System.out.println("caught" + e); } } }
finally clause
A finally keyword is used to create a block of code that follows a try block. A finally block of code always executes whether or not exception has occurred. Using a finally block, lets you run any cleanup type statements that you want to execute, no matter what happens in the protected code. A finally block appears at the end of catch block.
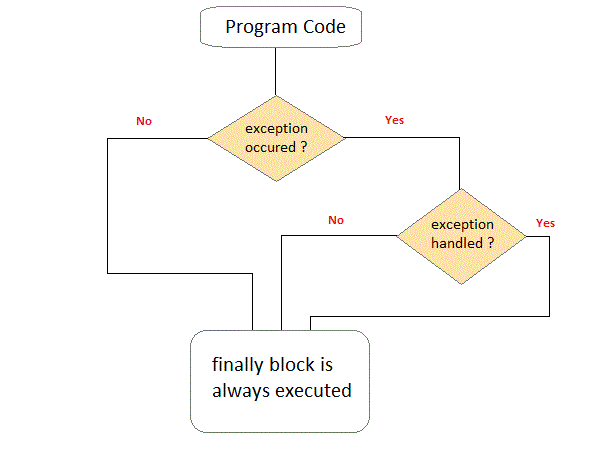
Example demonstrating finally Clause
Class ExceptionTest { public static void main(String[] args) { int a[]= new int[2]; System.out.println("out of try"); try { System.out.println("Access invalid element"+ a[3]); /* the above statement will throw ArrayIndexOutOfBoundException */ } finally { System.out.println("finally is always executed."); } } }
Output :
Out of try finally is always executed. Exception in thread main java. Lang. exception array Index out of bound exception.
You can see in above example even if exception is thrown by the program, which is not handled by catch block, still finally block will get executed.
Java try with resource statement ~ mkniit
JDK 7 introduces a new version of try statement known as try-with-resources statement. This feature add another way to exception handling with resources management,it is also referred to as automatic resource management.
Syntax
try(resource-specification) { //use the resource }catch() {...}
This try statement contains a paranthesis in which one or more resources is declare. Any object that implements
java.lang.AutoCloseable
or java.io.Closeable
, can be passed as a parameter to try statement. A resource is an object that is used in program and must be closed after the program is finished. The try-with-resources statement ensures that each resource is closed at the end of the statement, you do not have to explicitly close the resources.Java try and catch block ~ mkniit
Exception Handling Mechanism
In java, exception handling is done using five keywords,
- try
- catch
- throw
- throws
- finally
Exception handling is done by transferring the execution of a program to an appropriate exception handler when exception occurs.
Java Exception Handling ~ mkniit
Exception Handling is the mechanism to handle runtime malfunctions. We need to handle such exceptions to prevent abrupt termination of program. The term exception means exceptional condition, it is a problem that may arise during the execution of program. A bunch of things can lead to exceptions, including programmer error, hardware failures, files that need to be opened cannot be found, resource exhaustion etc.
Exception
A Java Exception is an object that describes the exception that occurs in a program. When an exceptional events occurs in java, an exception is said to be thrown. The code that's responsible for doing something about the exception is called an exception handler.
Saturday 13 May 2017
StringBuilder class ~ mkniit
StringBuilder is identical to StringBuffer except for one important difference it is not synchronized, which means it is not thread safe. Its because StringBuilder methods are not synchronised.
StringBuilder Constructors
- StringBuilder ( ), creates an empty StringBuilder and reserves room for 16 characters.
- StringBuilder ( int size ), create an empty string and takes an integer argument to set capacity of the buffer.
- StringBuilder ( String str ), create a StringBuilder object and initialize it with string str.
StringBuffer class ~ mkniit
StringBuffer class is used to create a mutable string object. It represents growable and writable character sequence. As we know that String objects are immutable, so if we do a lot of changes with String objects, we will end up with a lot of memory leak.
So StringBuffer class is used when we have to make lot of modifications to our string. It is also thread safe i.e multiple threads cannot access it simultaneously. StringBuffer defines 4 constructors. They are,
- StringBuffer ( )
- StringBuffer ( int size )
- StringBuffer ( String str )
- StringBuffer ( charSequence [ ]ch )
StringBuffer()
creates an empty string buffer and reserves room for 16 characters.stringBuffer(int size)
creates an empty string and takes an integer argument to set capacity of the buffer.
String class Fucntions ~ mkniit
The following methods are some of the most commonly used methods of String class.
charAt()
charAt() function returns the character located at the specified index.
String str = "studytonight"; System.out.println(str.charAt(2));
Output : u
equalsIgnoreCase()
equalsIgnoreCase() determines the equality of two Strings, ignoring thier case (upper or lower case doesn't matters with this fuction ).
String str = "java"; System.out.println(str.equalsIgnoreCase("JAVA"));
Output : true
Introduction to String ~ mkniit
Introduction to String Handling
String is probably the most commonly used class in java library. String class is encapsulated under
java.lang
package. In java, every string that you create is actually an object of type String. One important thing to notice about string object is that string objects are immutable that means once a string object is created it cannot be altered.What is an Immutable object?
An object whose state cannot be changed after it is created is known as an Immutable object. String, Integer, Byte, Short, Float, Double and all other wrapper class's objects are immutable.
Interface ~ mkniit
Interface is a pure abstract class.They are syntactically similar to classes, but you cannot create instance of an Interface and their methods are declared without any body. Interface is used to achieve complete abstraction in Java. When you create an interface it defines what a class can do without saying anything about how the class will do it.
Syntax :
interface interface_name { }
Example of Interface
interface Moveable { int AVERAGE-SPEED=40; void move(); }
Abstract class ~ mkniit
If a class contain any abstract method then the class is declared as abstract class. An abstract class is never instantiated. It is used to provide abstraction. Although it does not provide 100% abstraction because it can also have concrete method.
Syntax :
abstract class class_name { }
Abstract method
Method that are declared without any body within an abstract class are called abstract method. The method body will be defined by its subclass. Abstract method can never be final and static. Any class that extends an abstract class must implement all the abstract methods declared by the super class.
Syntax :
abstract return_type function_name (); // No definition
Package ~ mkniit
Package are used in Java, in-order to avoid name conflicts and to control access of class, interface and enumeration etc. A package can be defined as a group of similar types of classes, interface, enumeration and sub-package. Using package it becomes easier to locate the related classes.
Package are categorized into two forms
- Built-in Package:-Existing Java package for example
java.lang
,java.util
etc. - User-defined-package:- Java package created by user to categorized classes and interface
Command line Argument ~ mkniit
Command line argument in Java
The command line argument is the argument passed to a program at the time when you run it. To access the command-line argument inside a java program is quite easy, they are stored as string in String array passed to the args parameter of
main()
method.
Example
class cmd { public static void main(String[] args) { for(int i=0;i< args.length;i++) { System.out.println(args[i]); } } }
Output :
10 20 30
instanceof Operator ~ mkniit
In Java,
instanceof
operator is used to check the type of an object at runtime. It is the means by which your program can obtain run-time type information about an object. instanceof
operator is also important in case of casting object at runtime. instanceof
operator return boolean value, if an object reference is of specified type then it return true otherwise false.
Example of instanceOf
public class Test { public static void main(String[] args) { Test t= new Test(); System.out.println(t instanceof Test); } }
true
Monday 8 May 2017
Runtime Polymorphism ~ mkniit
Runtime Polymorphism or Dynamic method dispatch
Dynamic method dispatch is a mechanism by which a call to an overridden method is resolved at runtime. This is how java implements runtime polymorphism. When an overridden method is called by a reference, java determines which version of that method to execute based on the type of object it refer to. In simple words the type of object which it referred determines which version of overridden method will be called.
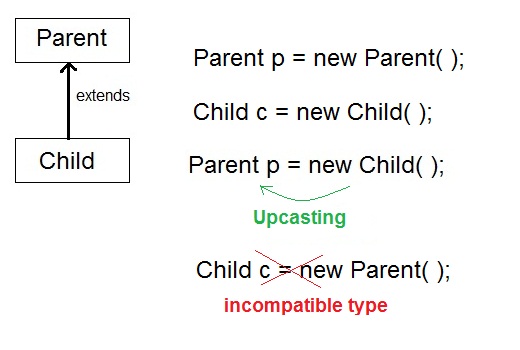
Method Overriding ~ mkniit
When a method in a sub class has same name and type signature as a method in its super class, then the method is known as overridden method. Method overriding is also referred to as runtime polymorphism. The key benefit of overriding is the abitility to define method that's specific to a particular subclass type.
Example of Method Overriding
class Animal { public void eat() { System.out.println("Generic Animal eating"); } } class Dog extends Animal { public void eat() //eat() method overriden by Dog class. { System.out.println("Dog eat meat"); } }
Aggregation ~ mkniit
Aggregation (HAS-A)
HAS-A relationship is based on usage, rather than inheritance. In other words, class A has-a relationship with class B, if code in class A has a reference to an instance of class B.
Example
class Student { String name; Address ad; }
Here you can say that Student has-a Address.
Inheritance ~ mkniit
Inheritance (IS-A)
Inheritance is one of the key features of Object Oriented Programming. Inheritance provided mechanism that allowed a class to inherit property of another class. When a Class extends another class it inherits all non-private members including fields and methods. Inheritance in Java can be best understood in terms of Parent and Child relationship, also known as Super class(Parent) and Sub class(child) in Java language.
Inheritance defines is-a relationship between a Super class and its Sub class.
extends
and implements
keywords are used to describe inheritance in Java.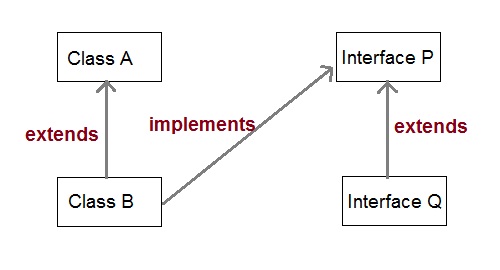
Subscribe to:
Posts (Atom)