How to write a Java program to multiply two matrices in Java is a very good programming exercise to get familiar with the two-dimensional array in Java. this example teaches about how to multiply arrays, how to access elements from a multi-dimensional array, how to pass them to a function etc. Since the matrix is a natural representation of multi-dimensional array in Java, they are often used to illustrate real word matrix exercises e.g. the calculating sum of two matrices or calculating the difference of two matrices etc. By the way, before writing the program, let's recap how to multiply two matrices in mathematics first. If you remember, you can only multiply two matrices if, and only if, the number of columns in the first matrix equals the number of rows in the second matrix. That is known as matrix multiplication criterion.
All Types GNIIT/NIIT Solution (Mr Jerry) Follow me on G+ and Twitter for more updates Twitter @mrjerry0001 #Write for us and get paid contact us On hike App:- @mrjerry4968
Pages
- Home
- HTML LAB@HOME
- DSA LAB@HOME
- WCD LAB@HOME
- C# LAB@HOME
- RDBMS LAB@HOME
- IADRT Lab@home
- EBDD LAB@HOME
- ACPC Lab@home
- LBEPS Lab@home
- ICTWC LAB@HOME
- SQL LAB@HOME
- WINDOWS APP LAB@HOME
- JAVA LAB@HOME
- NETWORK ESSENTIALS + LAB@HOME
- ASP.NET LAB@HOME
- LBEPS CYCLE TEST
- ASP.NET CYCLE TEST
- JAVA CYCLE TEST
- RDBMS CYCLE TEST
- DSA CYCLE TEST
- NIIT-HTML CYCLE TEST
- NIIT ASSIGNMENTS
- NIIT PROJECTS
- CORE JAVA NOTES
- NIIT BOOKS
- NIIT MT SOLVED
Friday 21 April 2017
10 Examples of CUT command in UNIX and Linux ~ mkniit
The cut command in UNIX is a nice utility program which allows you to cut data from a text file. The Linux cut command allows you to cut data by character, by field or by column. if used correctly along with sed, find, or grep in UNIX, the cut can do lots of reporting stuff. For example, you can extract columns from a comma separated file or a pipe or colon delimited file using cut command. For example, if you are only interested in first two columns you can show them using this command. In this Linux cut command tutorial we will see different options of cut command, different examples of Linux cut command and some important points about cut in UNIX.
In order to demonstrate the power of cut command through various examples, we will following colon delimited text file as input. This text file contains details of popular smartphones in 2011. The file contains 5 columns i.e. model, company, price, camera, and 4G in the same order.
In order to demonstrate the power of cut command through various examples, we will following colon delimited text file as input. This text file contains details of popular smartphones in 2011. The file contains 5 columns i.e. model, company, price, camera, and 4G in the same order.
Top 5 books to Learn Object Oriented Programming - Must Read, Best of Lot ~ mkniit
The OOP or Object Oriented Programming is one of the most popular programming paradigms which helps you to organize code in re the l world system. It's a tool which helps you to write complex software by thinking in terms of objects. Unlike its predecessor procedural programming paradigm which is implemented most notably by C, which solves the problem and complete task by writing code for computers, OOP style of programming allows you to think in terms of real world objects which has both state and behavior. You can view anything as objects and then find their state and behaviors, this will help you to simulate that object in code.
Unfortunately, programmers don't learn OOP or Procedural or Functional programming, what they learn is a programming language and as a side effect of that, they learn these paradigms. Since many developers learn Java, C++, or Python they learn OOP, but not in the true sense, hence a college graduate struggle to apply common OOP concepts in practice.
Unfortunately, programmers don't learn OOP or Procedural or Functional programming, what they learn is a programming language and as a side effect of that, they learn these paradigms. Since many developers learn Java, C++, or Python they learn OOP, but not in the true sense, hence a college graduate struggle to apply common OOP concepts in practice.
How to compare two XML files in Java - XMLUnit Example ~ mkniit
The XMLUnit library can be used to compare two XML files in Java. Similar to JUnit, XMLUnit can also be used to test XML files for comparison by extending the XMLTestcase class. It is a rich library and provides a detailed comparison of XML files. Btw, comparing XML is completely different than comparing String in Java or comparing object using equals(), as two XML which contains different comment and whitespace can be equals, which is not true for String or character comparison. Also while comparing XML files, it's very important to know exactly which content or part is different and XMLUnit not only shows the content which is different but also XPath of elements which is getting compared.
The heart and soul of XMLUnit are the DifferenceEngine class but we won't use it directly. Instead, you will use Diff and DetailedDiff the two important classes for XML comparison. They provide comparison engine for comparing XML. The XMLUnit library not only compares complete XML document but also can perform a lot of useful activities related to XPath e.g. it can check if an XPATH exists or not exists. It can even check if XPath contains expected value or not.
The heart and soul of XMLUnit are the DifferenceEngine class but we won't use it directly. Instead, you will use Diff and DetailedDiff the two important classes for XML comparison. They provide comparison engine for comparing XML. The XMLUnit library not only compares complete XML document but also can perform a lot of useful activities related to XPath e.g. it can check if an XPATH exists or not exists. It can even check if XPath contains expected value or not.
Difference between Abstraction and Encapsulation in Java - OOP ~ mkniit
Both Abstraction and Encapsulation are two of the four basic OOP concepts which allow you to model real-world things into objects so that you can implement them in your program and code. Many beginners get confused between Abstraction and Encapsulation because they both look very similar. If you ask someone what is Abstraction, he will tell that it's an OOP concept which focuses on relevant information by hiding unnecessary detail, and when you ask about Encapsulation, many will tell that it's another OOP concept which hides data from outside world. The definitions are not wrong as both Abstraction and Encapsulation does hide something, but the key difference is on intent.
Abstraction hides complexity by giving you a more abstract picture, a sort of 10,000 feet view, while Encapsulation hides internal working so that you can change it later. In other words, Abstraction hides details at the design level, while Encapsulation hides details at the implementation level.
For example, when you first describe an object, you talk in more abstract term e.g. a Vehicle which can move, you don't tell how Vehicle will move, whether it will move by using tires or it will fly or it will sell. It just moves. This is called Abstraction. We are talking about a most essential thing, which is moving, rather than focusing on details like moving in plane, sky, or water.
There are also the different levels of Abstraction and it's good practice that classes should interact with other classes with the same level of abstraction or higher level of abstraction. As you increase the level of Abstraction, things start getting simpler and simpler because you leave out details.On the other hand, Encapsulation is all about implementation. Its sole purpose is to hide internal working of objects from outside world so that you can change it later without impacting outside clients.
For example, we have a HashMap which allows you to store the object using put() method and retrieve the object using the get() method. How HashMap implements this method (see here) is an internal detail of HashMap, the client only cares that put stores the object and get return it back, they are not concerned whether HashMap is using an array, how it is resolving the collision, whether it is using linked list or binary tree to store object landing on same bucket etc.
Because of Encapsulation, you can change the internal implementation of HashMap with ease without impacting clients who are using HashMap. For example, in Java 8, the java.util.HashMap changes its implementation to use a binary tree instead of LinkedList to store objects in the same bucket after a certain threshold (see here).
The client doesn't need to make any change to benefit from this code change because those details are not exposed to them. Had client knows about that i.e. somehow they can get the reference of the internal array from HashMap, it would not have been possible to change the implementation without impacting clients.
There are many design principles which are based on Abstraction e.g. "coding for interfaces then implementation" which helps you to write flexible code in Java or C++. The idea is that a class depend upon on an interface, a higher level of abstraction than the class, a lower level of abstraction. This result in flexible code which can work with any implementation of the interface.
For example, if you need HashMap, your class should depend upon Map instead of HashMap. Similarly, if you need ArrayList, make sure you should use the List. Thankfully, Uncle Bob has shared several such design principles on Clean Code, collectively known as SOLID design principles, which is something every OOP programmer must learn and understand.
1) The most important difference between Abstraction and Encapsulation is that Abstraction solves the problem at design level while Encapsulation solves it implementation level.
2) Abstraction is about hiding unwanted details while giving out most essential details, while Encapsulation means hiding the code and data into a single unit e.g. class or method to protect inner working of an object from outside world. In other words, Abstraction means extracting common details or generalizing things.
3) Abstraction lets you focus on what the object does instead of how it does, while Encapsulation means hiding the internal details of how an object works. When you keep internal working details private, you can change it later with a better method. The Head First Object Oriented Analysis and Design has some excellent examples of these OOP concepts, I suggest you read that book at least once to revisit OOP fundamentals.
4) Abstraction focus on outer lookout e.g. moving of vehicle while Encapsulation focuses on internal working or inner lookout e.g. how exactly the vehicle moves.
5) In Java, Abstraction is supported using interface and abstract class while Encapsulation is supported using access modifiers e.g. public, private and protected.
Here is a nice table highlighting key differences between Abstraction and Encapsulation in Object Oriented Programming:
That's all about the difference between Abstraction and Encapsulation in Java and OOP. I understand, they both seems very similar but as I said they are a totally different concept. Just remember that Abstraction solves the problem at design level while Encapsulation solves at the implementation level. Both are very important for an OOP programmer but sometimes it can be difficult to explain.
As I have said before, the best way to learn and master Object-Oriented programming is by writing code and reading code of others. The more you are exposed to code, the more you realize how these concepts work in practice. There are several design principles which are based in terms of Abstraction e.g. coding for interface rather than implementation which allows you to write flexible code.
Other OOP concept tutorials you may like
Abstraction hides complexity by giving you a more abstract picture, a sort of 10,000 feet view, while Encapsulation hides internal working so that you can change it later. In other words, Abstraction hides details at the design level, while Encapsulation hides details at the implementation level.
For example, when you first describe an object, you talk in more abstract term e.g. a Vehicle which can move, you don't tell how Vehicle will move, whether it will move by using tires or it will fly or it will sell. It just moves. This is called Abstraction. We are talking about a most essential thing, which is moving, rather than focusing on details like moving in plane, sky, or water.
There are also the different levels of Abstraction and it's good practice that classes should interact with other classes with the same level of abstraction or higher level of abstraction. As you increase the level of Abstraction, things start getting simpler and simpler because you leave out details.On the other hand, Encapsulation is all about implementation. Its sole purpose is to hide internal working of objects from outside world so that you can change it later without impacting outside clients.
For example, we have a HashMap which allows you to store the object using put() method and retrieve the object using the get() method. How HashMap implements this method (see here) is an internal detail of HashMap, the client only cares that put stores the object and get return it back, they are not concerned whether HashMap is using an array, how it is resolving the collision, whether it is using linked list or binary tree to store object landing on same bucket etc.
Because of Encapsulation, you can change the internal implementation of HashMap with ease without impacting clients who are using HashMap. For example, in Java 8, the java.util.HashMap changes its implementation to use a binary tree instead of LinkedList to store objects in the same bucket after a certain threshold (see here).
The client doesn't need to make any change to benefit from this code change because those details are not exposed to them. Had client knows about that i.e. somehow they can get the reference of the internal array from HashMap, it would not have been possible to change the implementation without impacting clients.
There are many design principles which are based on Abstraction e.g. "coding for interfaces then implementation" which helps you to write flexible code in Java or C++. The idea is that a class depend upon on an interface, a higher level of abstraction than the class, a lower level of abstraction. This result in flexible code which can work with any implementation of the interface.
For example, if you need HashMap, your class should depend upon Map instead of HashMap. Similarly, if you need ArrayList, make sure you should use the List. Thankfully, Uncle Bob has shared several such design principles on Clean Code, collectively known as SOLID design principles, which is something every OOP programmer must learn and understand.
Difference between Abstraction vs Encapsulation
Here are some of the key difference between Encapsulation and Abstraction in point format for your quick revision:1) The most important difference between Abstraction and Encapsulation is that Abstraction solves the problem at design level while Encapsulation solves it implementation level.
2) Abstraction is about hiding unwanted details while giving out most essential details, while Encapsulation means hiding the code and data into a single unit e.g. class or method to protect inner working of an object from outside world. In other words, Abstraction means extracting common details or generalizing things.
3) Abstraction lets you focus on what the object does instead of how it does, while Encapsulation means hiding the internal details of how an object works. When you keep internal working details private, you can change it later with a better method. The Head First Object Oriented Analysis and Design has some excellent examples of these OOP concepts, I suggest you read that book at least once to revisit OOP fundamentals.
4) Abstraction focus on outer lookout e.g. moving of vehicle while Encapsulation focuses on internal working or inner lookout e.g. how exactly the vehicle moves.
5) In Java, Abstraction is supported using interface and abstract class while Encapsulation is supported using access modifiers e.g. public, private and protected.
Here is a nice table highlighting key differences between Abstraction and Encapsulation in Object Oriented Programming:
That's all about the difference between Abstraction and Encapsulation in Java and OOP. I understand, they both seems very similar but as I said they are a totally different concept. Just remember that Abstraction solves the problem at design level while Encapsulation solves at the implementation level. Both are very important for an OOP programmer but sometimes it can be difficult to explain.
As I have said before, the best way to learn and master Object-Oriented programming is by writing code and reading code of others. The more you are exposed to code, the more you realize how these concepts work in practice. There are several design principles which are based in terms of Abstraction e.g. coding for interface rather than implementation which allows you to write flexible code.
Other OOP concept tutorials you may like
- 5 Books to Learn Object-Oriented Programming and Design (book)
- What is the difference between Class and Object in Java or OOP? (answer)
- The difference between Inheritance and Polymorphism in Java? (answer)
- The difference between Aggregation, Composition, and Association in OOP? (answer)
- The difference between State and Strategy design patterns? (answer)
- What is Polymorphism in Java? Overloading or Overriding? (answer)
- What is the difference between Overloading and Overriding in OOP? (answer)
- The difference between instance and object in Java? (answer)
- What is the difference between static and dynamic binding in Java? (answer)
Difference between ArrayList and ArrayList> in Java - Raw Type vs Wildcard ~ mkniit
One of my readers asked me about the difference between ArrayList vs ArrayList< in Java?>, which was actually asked to him on a recent Java development interview. The key difference between them is that ArrayList is not using generics while ArrayList is a generic ArrayList but they looks very similar. If a method accepts ArrayList or ArrayList<?> as a parameter then it can accept any type of ArrayList e.g. ArrayList of String, Integer, Date, or Object, but if you look closely you will find that one is raw type while other is using an unbounded wildcard. What difference that could make? Well, that makes a significant difference because ArrayList with raw type is not type safe but ArrayList<?> with the unbounded wildcard is type safe.
Log4j Tips : Use MDC or Mapped Dignostic Context to distinguish logging per Client or Request ~ mkniit
The MDC or Mapped Diagnostic Context is a concept or feature of Log4j logging library which can be used to group related log messages together. For example, by using MDC you can stamp a unique identification String like clientId or orderId on each log message and then by using grep command in Linux, you can extract all log messages for a particular client or order to understand exactly what happened to a particular order. This is especially very useful in multi-threaded, concurrent Java applications where multiple threads are simultaneously processing multiple orders from multiple clients. In such applications, searching for relevant log messages in a big log file where log messages for multiple orders or clients are overlapping is a big task.
The MDC concept is not a new concept and available since log4j 1.2 version. Since most of real world systems e.g. high-frequency trading application process thousands of order simultaneously where different thread runs the same piece of code but with different data, each execution is unique, but without a unique identification it's difficult to find all log messages related to processing a particular order. The MDC or Mapped Diagnostic Context feature of Log4j solves that problem by stamping unique identification string on relevant log messages.
The MDC concept is not a new concept and available since log4j 1.2 version. Since most of real world systems e.g. high-frequency trading application process thousands of order simultaneously where different thread runs the same piece of code but with different data, each execution is unique, but without a unique identification it's difficult to find all log messages related to processing a particular order. The MDC or Mapped Diagnostic Context feature of Log4j solves that problem by stamping unique identification string on relevant log messages.
Can we declare a class Static in Java? ~ mkniit
The answer to this question is both Yes and No, depending on whether you are talking about a top level class or a nested class in Java. You cannot make a top level class static in Java, the compiler will not allow it, but you can make a nested class static in Java. A top level class is a class which is not inside another class. It may or may not be public i.e. you can have more than one class in a Java source file and only needs to be public, whose name must be same as the name of the file, rest of the class or interface on that file may or may not be public. On the other hand, a nested class is a class inside a top level class. It is also known as the inner class or member class in Java.
Saturday 15 April 2017
Download professional Asp.Net 4.5 in C# book pdf free ~ mkniit ~ gniitsolution
The all-new approach for experienced ASP.NET professionals!
ASP.NET is Microsoft’s technology for building dynamically generated web pages from database content. Originally introduced in 2002, ASP.NET has undergone many changes in multiple versions and iterations as developers have gained a decade of experience with this popular technology. With that decade of experience, this edition of the book presents a fresh, new overhauled approach.
- A new focus on how to build ASP.NET sites and applications relying on field-tested reliable methods
- Integration of “One ASP.NET” philosophy treating ASP.NET Web Forms and ASP.NET MVC as equal tools each with their proper time and place
- Coverage of hot new ASP.NET 4.5 additions such as the Web API, Websockets and HTML5 & CSS3 use in layout but only to the extent that the tools themselves are practical and useful for working ASP.NET developers
C# NULLABLES ~ mkniit
C# NULLABLES
DefinitionNullablle type is a data type containing a defined data type or a null value.
You should note here that here the variable datatype has been awarded and then only may be used.
The concept of a nullable type are not compatible with “var”.
I will explain this with the syntax in the next section.
Declaration
Any datatype can be declared nullable types with the help of the “?” operator.
An example of the syntax is as follows:
C# NAMESPACES ~ mkniit
C# NAMESPACES
Namespace is a set of retaining the name provides a way to separate the other is designed for. The name of the class that is declared in a namespace declared in the same class name does not conflict with another.Define a Namespace
Namespace definition keywords namespace namespace name is followed by the following:
Call it this version is activated by the function or variable namespace, add the name of the namespace as follows:
C# SWITCH CASE STATEMENT ~ mkniit
C# SWITCH CASE STATEMENT
Switch Case statement is also one conditioned statements. Pernyatan Switch Case is basically almost the same as the if then else. If you require a statement with many conditions, use the Switch Case statement. For more mamahami about the Switch Case statement, follow the following steps-steps:
1. Create a new application project. In Visual Studio, on the menu click File> New >Project. For more details, see the following menu on the display.
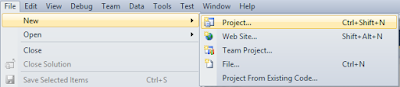
2. Then will appear the window New Project like the look below.
CSHARP OPERATOR OVERLOADING ~ mkniit
C# OPERATOR OVERLOADING
You can redefine or excessive bulk of the built-in operators can be used in c #. So that a programmer can use operators and user-defined types. Overloaded operator is a special operator name keywords followed by the symbols for the specified operator function. Similar to other functions, overloaded operators have a type argument list, and return.To cite an example, go through the following features:
The function above the addition operator (+) does not apply to user-defined classes box. It adds two properties to the object box, and returns the resulting box objects.
CSHARP VARIABLE AND DATA TYPES ~ mkniit
C# VARIABLE AND DATA TYPES
Before learning a programming technique, you must first master the programming language in question. Let's say you want to learn C # programming, you must learn first how to communicate with C # programmingenvironment that is by understanding the basics – and understand basic programming languages in use in C#.Data Types In C #
In C # there are two types of data, namely:
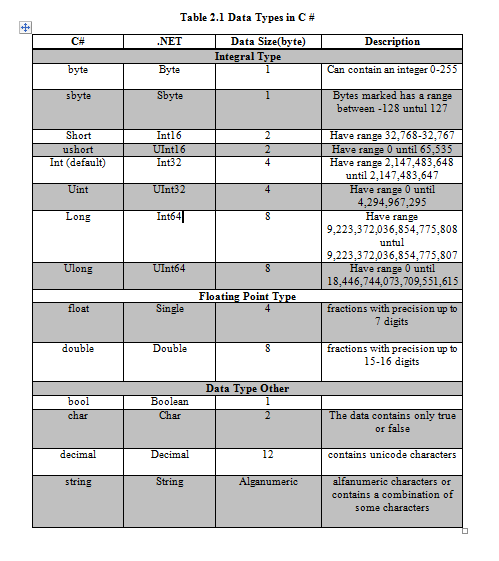
Subscribe to:
Posts (Atom)